VDD Charge Analysis module#
When a calculation is performed and has succesfully finished, the VDD charge analysis module can be used to extract the Voronoi Deformation Density (VDD) charges. The VDD charges measure the charge flow between atoms (officially Voronoi cells) from non-interacting atoms (“promolecule”) to atoms feeling the environment of other atoms (molecular enviroment). If symmetry is used, the VDD charges can be decomposed into contributions of seperate irreducible representations.
Requirements#
A finished calculation using the ADF engine.
Location to the calculation directory with ams.rkf and adf.rkf files
Example#
First, relevant modules need to be loaded. These include pathlib for handling paths and tcutility.results for loading the results of the calculation. In addition, the VDD manager module needs to be loaded which is the interface to performing VDD analyses.
Note
For this example we use calculations found in the VDD test directory of the tcutility package. We assume that the you place the VDD test directory in the same directory as this script. See the example python script for a direct implementation
import pathlib as pl
import tcutility.results as results
from tcutility.analysis.vdd import manager
Now, the calculation directory needs to be specified and the calculation results can be loaded via the read
function.
The next step is to create a VDDManager
object which is the interface to performing VDD analyses.
DIRS = {0: "fa_acid_amide_cs", 1: "fa_squaramide_se_cs", 2: "fa_donor_acceptor_nosym", 3: "geo_nosym"}
# Specify the calculation directory
base_dir = pl.Path(__file__).parent
base_dir = base_dir / "VDD"
calc_dir = base_dir / DIRS[1]
calc_res = results.read(calc_dir)
vdd_manager = manager.create_vdd_charge_manager(name=calc_dir.name, results=calc_res)
The VDDManager
object contains the total VDD charges, as well as the VDD charges for each irreducible representation.
To see the contents of the VDDManager
, simply print it. The charges are formatted as a table in the unit of milli electrons [me].
You can always change the unit of the charges by using the change_unit
method.
vdd_manager.change_unit("me") # Change the unit to electrons "e" (electrons) or "me" (milli electrons)
# Print the VDD charges to standard output
print(vdd_manager)
# Output:
fa_squaramide_se_cs
VDD charges (in unit me):
Frag Atom Total AA AAA
1 1C -40 -10 -30
1 2Se -165 -30 -134
1 3C -40 -10 -30
1 4Se -165 -30 -134
1 5C +26 +122 -96
1 6C +26 +122 -96
2 7N +62 -88 +150
2 8H +53 -2 +55
2 9H +63 +7 +56
2 10N +62 -88 +150
2 11H +63 +7 +56
2 12H +53 -2 +55
Summed VDD charges (in unit me):
Frag Total AA AAA
1 -357 +164 -521
2 +357 -164 +521
See? It is that easy. The charges sum up to the total charge of the molecule (in this case 0). Also, the sum of irrep charges is equal to the total charge, as it should be. It is possible to print the charges to both a text file (.txt) as well as a Excel (.xlsx) file. The Excel file is formatted as a table and can be used for further analysis.
output_dir = base_dir / "output"
# Write the VDD charges to a text file (static method because multiple managers can be written to the same file)
manager.VDDChargeManager.write_to_txt(output_dir, vdd_manager)
# Write the VDD charges to an excel file
vdd_manager.write_to_excel(output_dir)
To visualize the VDD charges further, the charge for each atom can be plotted in a bar graph using matplotlib. The plot is saved as a .png file.:
# Plot the VDD charges per atom in a bar graph
vdd_manager.plot_vdd_charges_per_atom(output_dir)
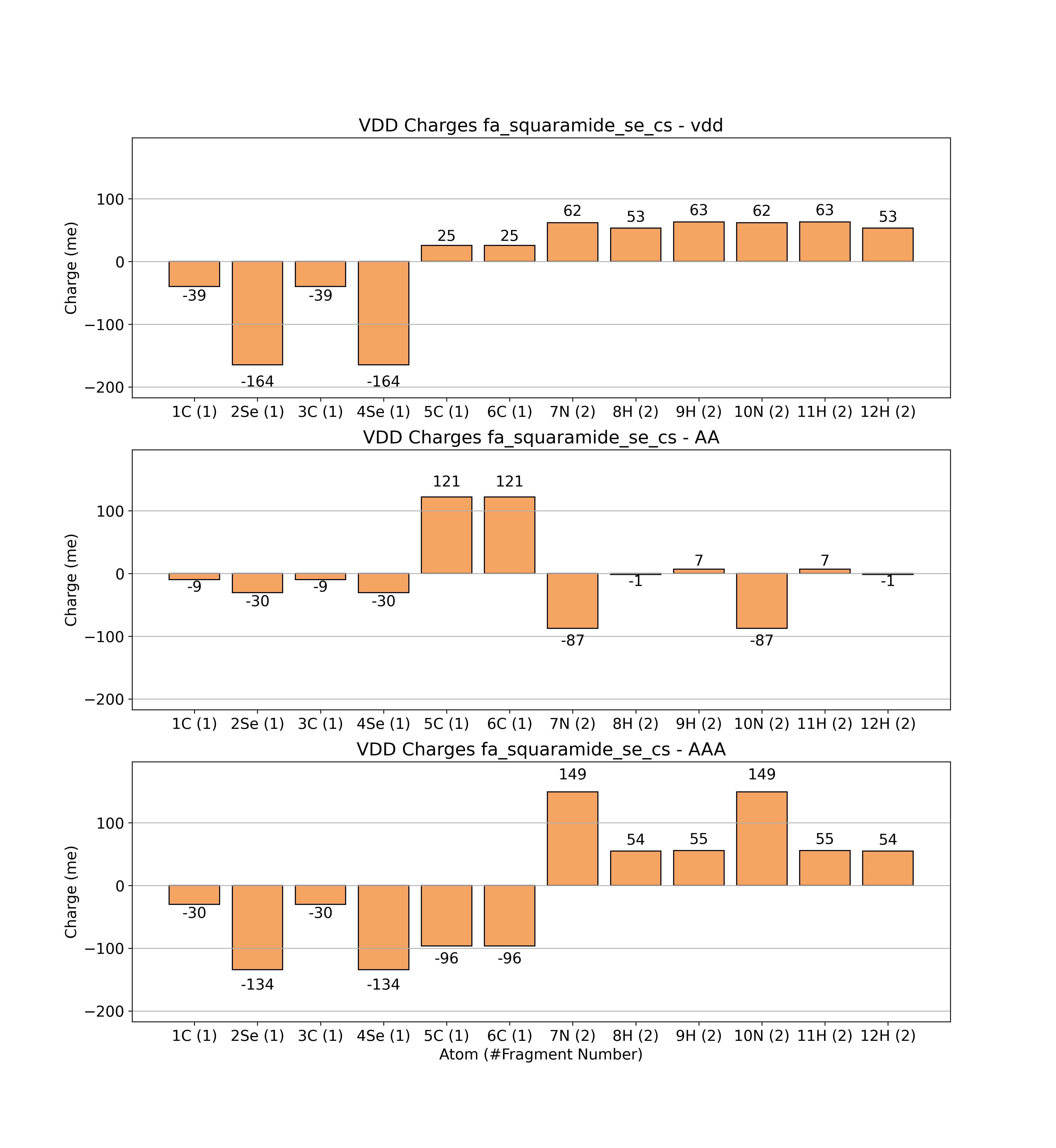
Finally, multiple VDDManager
objects can be made and written to a single .txt file. This is useful when comparing multiple calculations.
# Multiple calculations can be combined into a single file
calc_dirs = [base_dir / calc for calc in DIRS.values()]
calc_res = [results.read(calc_dir) for calc_dir in calc_dirs]
vdd_managers = [manager.create_vdd_charge_manager(name=calc_dir.name, results=res) for calc_dir, res in zip(calc_dirs, calc_res)]
manager.VDDChargeManager.write_to_txt(output_dir, vdd_managers)
VDD charges (in unit me):
fa_acid_amide_cs
Frag Atom Total AA AAA
1 1C +19 +1 +18
1 2O -8 -49 +41
1 3Se -68 -5 -63
2 4O -34 +27 -61
2 5C +10 +3 +8
2 6H +6 +5 +1
2 7H +2 -2 +4
2 8H +44 +41 +3
2 9N -4 -46 +42
1 10H +32 +31 +1
1 11H +1 -6 +6
fa_squaramide_se_cs
Frag Atom Total AA AAA
1 1C -40 -10 -30
1 2Se -165 -30 -134
1 3C -40 -10 -30
1 4Se -165 -30 -134
1 5C +26 +122 -96
1 6C +26 +122 -96
2 7N +62 -88 +150
2 8H +53 -2 +55
2 9H +63 +7 +56
2 10N +62 -88 +150
2 11H +63 +7 +56
2 12H +53 -2 +55
fa_donor_acceptor_nosym
Frag Atom Total
1 1C +0
1 2C +0
1 3C -0
1 4C -0
1 5H +1
1 6H +1
1 7H +1
1 8H +0
1 9H +1
1 10H +2
1 11H +1
2 12O -1
1 13O -2
1 14N -13
2 15H +6
2 16H -7
2 17H -9
1 18H +20
geo_nosym
Frag Atom Total
1 1C -105
5 2H +67
11 3H +68
16 4H +55
17 5C +166
18 6O -291
19 7N -116
20 8H +158
21 9N -84
2 10H +165
3 11C +213
4 12O -253
8 13N -235
10 14H +54
14 15H +59
15 16C +45
22 17C -68
23 18C -50
24 19C -59
25 20C -53
6 21C -64
12 22H +65
7 23H +52
9 24H +48
13 25H +164
VDD analysis API#
Here is the implementation of the VDDManager
and VDDCharge
classes. The central information contained in the VDDManager
is a dictionary containing the total charge, and irreps (if present) as keys and the a list of VDDCharge
as value.
A VDDCharge
does not only contain the charge itself, but also to which atom and frag index it belongs.